Last updated: 2023-01-21
Using Lombok in a Spring Boot project with IntelliJ
Did you know that you can save days or weeks of development time when starting new Spring Boot apps? br With Bootify you have the right helper at your side - get a runnable prototype in minutes and focus on your business logic instead. Best practices included.
Discover more
Lombok is no ordinary Java library - Lombok provides annotations which generate Java code on the fly. This can drastically shorten the source code which has to be written.
Preparation
To use Lombok in our project, first the dependency must be included in the build.gradle
or pom.xml
. Here the annotation processor is especially important, so that Lombok can intervene directly in the compilation process. Bootify adds these dependencies automatically to the project if Lombok is enabled.
Lombok dependencies in the build.gradle
Now Lombok must be activated explicitly in the IDE. In IntelliJ you have to install a plugin for that.
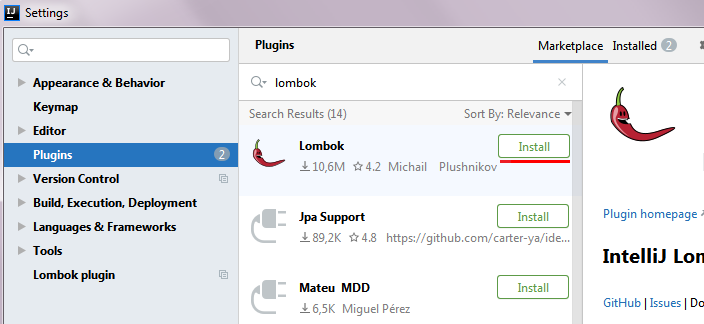
Installation of the Lombok plugin in IntelliJ under "Settings -> Plugins"
Furthermore, annotation proceccing must be activated. This is already included in the dependencies, but is only used by Gradle and Maven there. IntelliJ uses its own build process, which has to be adapted separately. The newest version of IntelliJ will provide a warning in case your forgot this.
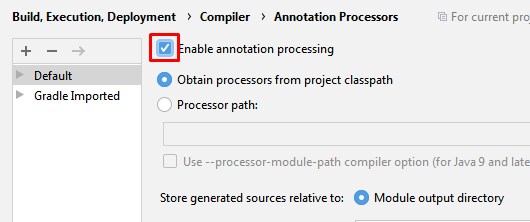
Activate Annotation Processing under Settings → Build, Execution, Deployment → Compiler → Annotation Processors
The most useful helpers
Here are a few examples of these great annotations, which can save a lot of boilerplate code.
As the names suggest, these annotations create getters and setters for all fields
With @Slf4j a static field "log" is added to the class without extra coding
@SneakyThrows is hiding exceptions from the developer
With @SneakyThrows
Lombok will hide the Exception that a developer would usually be required to catch with a try-catch
clause around the method. So the developer doesn't have to do this manually - in most cases a global exception handling is sufficient.
Other very practical annotations are @AllArgsConstructor
, @Builder
and @Value
- more information can be found in the following sources.
Further readings
Lombok features
Enable Lombok in Eclipse
Introduction to Lombok