Last updated: 2024-03-20
Integrating Keycloak with Angular and Spring Boot
Keycloak is an Open Source software and provides a wide range of options for user management and authentication. How can we integrate Keycloak into our application based on Angular and Spring Boot?
Backgrounds
There are different ways for authentication with Keycloak, but OAuth with OIDC is generally the first choice. If we are working with a single page application based on Angular, the basic process is as follows.
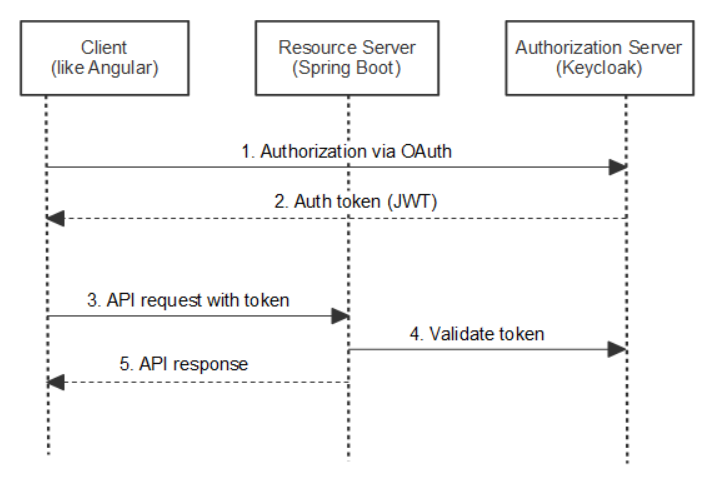
Accessing the resource server with a token
Our Angular client authenticates itself directly with Keycloak and receives a token as a result. This token is included in the requests to our Spring Boot backend, and our backend verifies the validity of the token using Spring Security.
How we set up our Spring Boot app as a resource server for Keycloak is described in this article. We can integrate Angular into our application on the basis of this article. After these steps, we should have a configured Keycloak and a Spring Boot application with Angular. The following section describes how to set up our client for OAuth.
Adding OAuth to our Angular client
Keycloak already provides a JavaScript library keycloak-js
. Many articles also refer to the keycloak-angular
library. However, this offers little added value, so we only add the first one to our project.
The AuthenticationService
will provide all functions related to authentication. Therefore, we first create this class with the init
function.
First version of our AuthenticationService
The creation of the required client within the Keycloak server has already been described here. We have to create a public client, as we cannot pass and store the secret in the browser. In addition, our Angular development server http://localhost:4200/
must be specified as the redirect URI.
Having our Keycloak server ready, we can prepare the matching configuration in our Angular client. The config is added to environment.ts
in order to distinguish between the development and production system later on.
Keycloak settings in our environment.development.ts
With the specified setting onLoad:'check-sso'
an existing authentication is automatically picked up. To do this, we have to create a file silent-check-sso.html
in the assets
folder. More details can be found in the documentation of the adapter.
Creating silent-check-sso.html
Now we can call the init method of our service during the application start. The singleton is passed to our factory method as a dependency.
Extension of our app.config.ts
This should start our Angular client without errors, although we are not yet using Keycloak functionalities in any way. Let's change that now.
Providing login and logout
In order to add the actual login and logout, we first extend our AuthenticationService
with the following methods.
Adding new methods to our AuthenticationService
This allows us to query the login status and initiate login and logout. The logout always leads back to the homepage. When logging in, we can include a targetUrl - the user is redirected there as soon as he has successfully authenticated himself. We can now add a reference authenticationService = inject(AuthenticationService);
to our HeaderComponent
and integrate the new functions into its template.
Calling login and logout on click
By clicking on the login button, the user is redirected to Keycloak and can authenticate himself there. The header then switches to the logout button.
Adding the token
Last but not least, we want to add the token to all outgoing requests to our Spring Boot backend. To do this, we create an AuthenticationInterceptor
that attaches the token as Bearer ...
to all API requests.
Including the token in all requests
As we want to wait for the token to be provided, we use the lastValueFrom
converter of rxjs
. Now we have to extend our app.config.ts
again.
The last thing missing is the getToken()
method. The tokens have a very short validity by default, so we use the option of automatically renewing expired tokens.
Renewing and returning the token
With this we have completely integrated Keycloak into our Angular client! When a user has successfully authenticated, the JWT is automatically sent along the request and our Spring Boot backend can ensure its validity.
In the Professional plan of Bootify, Keycloak Resource Server can be selected for the Spring Security setup. If Angular has also been selected for the frontend, the configuration described here is fully provided - individually tailored to your selected settings.
See Pricing
or read quickstart
Further readings
Angular environments
Keycloak JavaScript adapter